Schedule your Python code quickly with .serve()
Prefect has a simplified Python method for creating a deployment automatically
Sometimes, you just want to get a script scheduled very quickly. In this blog, we’ll cover how you can start scheduling your code by adding just a couple of lines to your .py file.
While it only takes minutes to get start, Prefect is built to be a powerful workflow orchestration tool that can accommodate a variety of complex scenarios:
- Running workflows on a variety of infrastructure (Kubernetes, Serverless, on distributed systems like Dask, etc.)
- Heavy customization
- Dynamic dependencies
- Event-driven workflows
- Complex security requirements
Get started with your first scheduled flow today, and soon you'll be building out custom workflows based on your own unique requirements.
Simple Python Transformation
Let's say that you have a process that you created in Python, that downloads some data and then transforms it. This could describe innumerable scenarios, so in this example let's calculate the moving average of a stock price.
1import yfinance as yf
2
3
4def create_moving_average():
5 # download data
6 data = yf.download(tickers='SNOW', period='10d', interval='1h')
7
8 # calculate moving average
9 sma = data["Close"].rolling(48).mean()
10
11 # print results
12 print(sma)
13
14
15if __name__ == "__main__":
16 create_moving_average()
The above script downloads 10 days worth of Snowflake's stock price on an hourly interval using the yfinance library. If you were to execute this file, it would calculate the rolling average.
What if I want to set this up to run on a schedule, automating my work so I don't need to manually run this every hour (or day, or week)?
.serve()
To get your code scheduled with Prefect, you could use the .serve() method right within your code.
First, log in to Prefect on your local environment by using prefect cloud login. Once you've done that, you can simply execute the code below.
1import yfinance as yf
2from prefect import flow
3
4
5@flow
6def create_moving_average():
7 # download data
8 data = yf.download(tickers='SNOW', period='10d', interval='1h')
9
10 # calculate moving average
11 sma = data["Close"].rolling(48).mean()
12
13 # print results
14 print(sma)
15
16
17if __name__ == "__main__":
18 create_moving_average.serve(name="moving_average_deployment",
19 interval=3600)
By adding the Prefect library, decorating our code with @flow, and adding the .serve method, we now have a a scheduled workflow that you can even see in the Prefect UI.
Check out your flow runs page, and you'll see your deployment has been created automatically! Deployments are a Prefect concept that allow the code in your flow to become scheduled. Your script is now scheduled on an hourly basis, and as long as your machine is on your flow will run as scheduled.
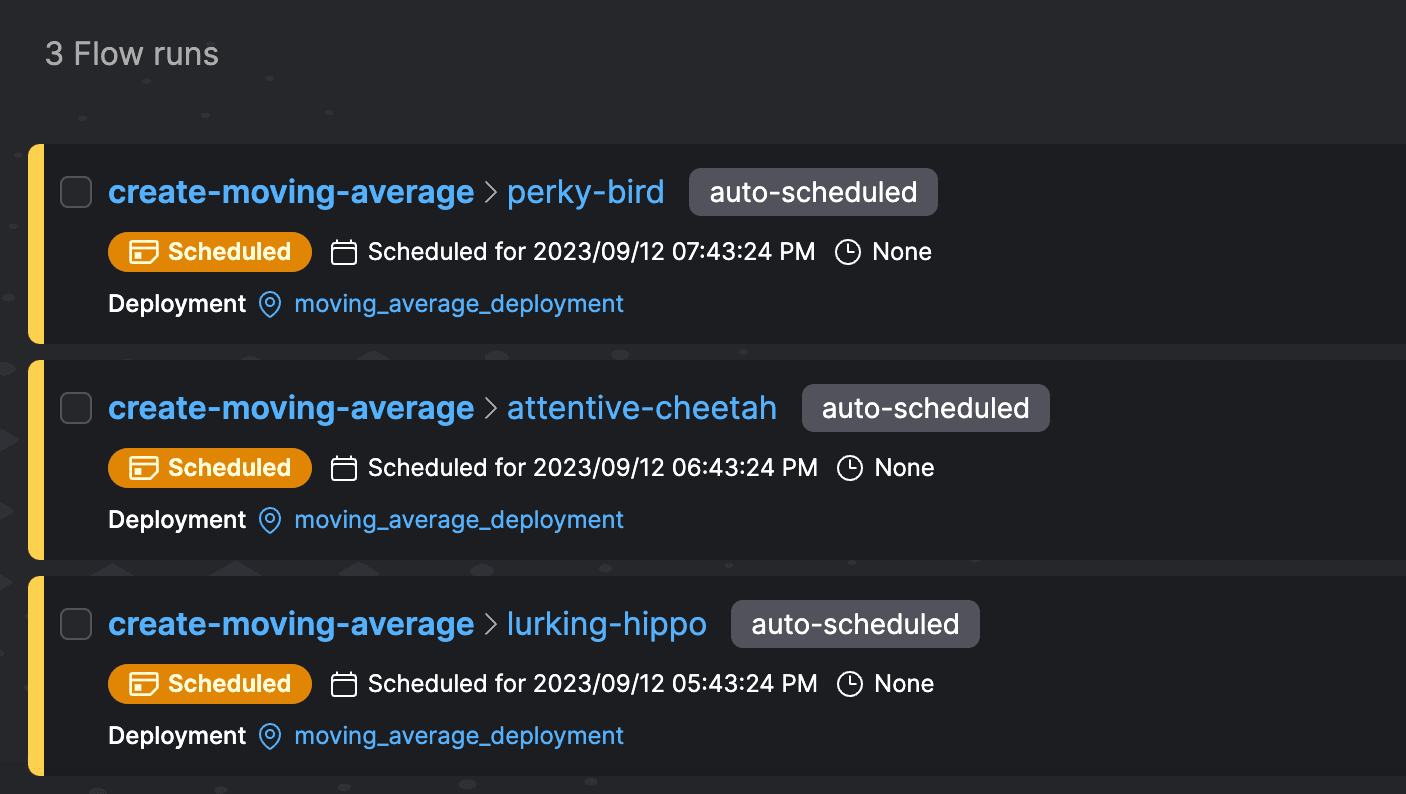
Note that deployments created with this method are executing the code on the machine where it is created. If you want to start defining other remote infrastructure, you'll need to start defining your own deployments.
Customizing Your Prefect Deployment
Congrats, you've got a flow scheduled with Prefect! A deployment was automatically created for you.
If you want to start configuring your workflows to run automatically based on your own complex criteria, you'll want to create your own deployments. Deployments are powerful objects that act as a server-side endpoint for a flow. They allow you to:
- schedule flows and trigger them remotely
- define where flows run, based on workers and work pools
- support paramaterization
Getting started with Prefect has never been easier than with the new .serve() method.
Prefect makes complex workflows simpler, not harder. Try Prefect Cloud for free for yourself, download our open source package, join our Slack community, or talk to one of our engineers to learn more.
Related Content








